How to use GraphQL Code Generator with Apollo
4 July 2022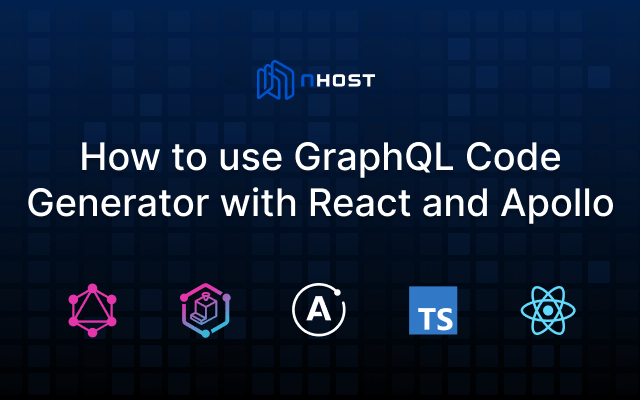
GraphQL can improve app development speed, but to fully leverage its potential, a GraphQL Code Generator (codegen) is necessary. In this blog post, discover how to use GraphQL Code Generator to enhance GraphQL development with React, Apollo Client, and TypeScript. By generating Typed Queries, Mutations, and Subscriptions, GraphQL Code Generator ensures accurate typings and autocompletion for all queries, mutations, and subscription variables and results in your code. Let's begin.
Setup
Setting up GraphQL Code Generator only takes three easy steps:
- Install the necessary npm packages.
- Create a configuration file for the GraphQL Code Generator.
- Include a script for the Code Generator in
package.json
.
Install NPM packages
Install the following npm packages required to run GraphQL Code Generator.
_10npm install graphql_10npm install -D typescript @graphql-codegen/cli @graphql-codegen/client-preset @graphql-typed-document-node/core_10# or yarn_10yarn add graphql_10yarn add -D typescript @graphql-codegen/cli @graphql-codegen/client-preset @graphql-typed-document-node/core_10# or pnpm_10pnpm add graphql_10pnpm add -D typescript @graphql-codegen/cli @graphql-codegen/client-preset @graphql-typed-document-node/core
Create a Config File for GraphQL Code Generator
Next, create a codegen.ts
configuration file next to your package.json
file.
This configuration file contains information about:
- GraphQL API URL.
- GraphQL API headers.
- Where GraphQL queries and mutations exist in your code.
Update the file with your GraphQL endpoint and headers. Here's an example:
_23import { CodegenConfig } from '@graphql-codegen/cli'_23_23const config: CodegenConfig = {_23 schema: [_23 {_23 'http://localhost:1337/v1/graphql': {_23 headers: {_23 'x-hasura-admin-secret': 'nhost-admin-secret',_23 },_23 },_23 },_23 ],_23 ignoreNoDocuments: true,_23 generates: {_23 './src/gql/': {_23 documents: ['src/**/*.tsx'],_23 preset: 'client',_23 plugins: [],_23 },_23 },_23}_23_23export default config
This example configuration file is for a Nhost project using Hasura. We've set the GraphQL endpoint to http://localhost:1337/v1/graphql
and added the x-hasura-admin-secret
header so the GraphQL Code Generator can introspect the GraphQL API as an admin to get all queries and mutations available.
GraphQL autocomplete in VS Code
This step is optional but highly recommended.
You can get autocomplete when writing GraphQL queries and mutations in VS Code. This is another great way to improve your development workflow and reduce errors caused by typos.
GraphQL inline autocomplete
To get inline autocomplete when you're writing GraphQL in VS Code, install the GraphQL for VSCode extension and add the following configuration (graphql.config.yaml
) file next to your package.json
file:
_10schema:_10 - http://localhost:1337/v1/graphql:_10 headers:_10 x-hasura-admin-secret: nhost-admin-secret
Add a Codegen Script to package.json
As the last step, add a script to the package.json
to run the GraphQL Code Generator.
_10{_10 /*...*/_10 "scripts": {_10 /*...*/_10 "codegen": "graphql-codegen"_10 }_10 /*...*/_10}
You'll run this script (npm run codegen
) every time you've changed anything in your GraphQL API or in your GraphQL files to get the most up-to-date types generated. You can also run the script in watch mode to automatically generate new types when you change your code using npm run codegen -w
.
You've now completed the three configuration steps. It's time to start using the GraphQL Code Generator and unleash its power!
Workflow
As a mental model, this is how you will use the GraphQL Code Generator in your development workflow:
- Run
npm run codegen -w
in watch mode to automatically generate types for queries, mutations, and subscriptions. - Write GraphQL queries and mutations and wrap the GraphQL code with the
graphql()
function. - Use the generated types to get autocompletion and type safety.
The graphql()
function is generated by the GraphQL Code Generator and located at src/gql/gql
.
Here's an example of how to write a GraphQL query and wrap it with the graphql()
function:
_11import { graphql } from '../gql/gql'_11_11const GET_TASKS = graphql(`_11 query GetTasks {_11 tasks(order_by: { createdAt: desc }) {_11 id_11 name_11 done_11 }_11 }_11`)
Example: Todo App
We'll now go through two examples of how to use the GraphQL Code Generator setup with Apollo Client in a Todo App. The first example is by using a query to fetch data and the other example is to use a mutation to modify data.
The full source code can be found on GitHub - GraphQL Code Generator Example with Apollo Client. This blog post contains a subset of the code to give you a basic understanding of how to use GraphQL Code Generator with Apollo Client.
For the two examples, we're using a table tasks
in Hasura with three columns:
- id - UUID
- name - Text
- done - Boolean
App Setup
Install Apollo Client (@apollo/client
). We'll also install @nhost/react
and @nhost/react-apollo
to get a pre-configured Apollo client and the NhostApolloProvider
component to wrap our app as well as some helper functions for Nhost and React. If you're not using Nhost, you can configure your own Apollo client and Apollo Provider following Apollo's getting started guide.
_10npm install @apollo/client @nhost/react @nhost/react-apollo_10# or yarn_10yarn add @apollo/client @nhost/react @nhost/react-apollo_10# or pnpm_10pnpm add @apollo/client @nhost/react @nhost/react-apollo
Let's continue by first setting up the Apollo client in our app.
App
In App.tsx
, we'll set up the Apollo client and wrap our app with the NhostApolloProvider
component. Again, if you're not using Nhost, you can set up your own Apollo client and Apollo Provider.
_24import { NhostApolloProvider } from '@nhost/react-apollo'_24import { NhostClient, NhostProvider } from '@nhost/react'_24_24import { Tasks } from './components/Tasks'_24_24const nhost = new NhostClient({_24 subdomain: '<app-subdomain>',_24 region: '<app-region>',_24})_24_24function App() {_24 return (_24 <NhostProvider nhost={nhost}>_24 <NhostApolloProvider nhost={nhost}>_24 <div>_24 <div>Todo App</div>_24 <Tasks />_24 </div>_24 </NhostApolloProvider>_24 </NhostProvider>_24 )_24}_24_24export default App
Get Tasks (GraphQL Query)
As we mentioned under the Workflow section above, we use the three-step process when using GraphQL Code Generator.
Just to repeat, these are the three steps:
- Run
npm run codegen -w
in watch mode to automatically generate types for GraphQL queries, mutations, and subscriptions. - Write GraphQL queries and mutations and wrap the GraphQL code with the
graphql()
function. - Use the generated types to get autocompletion and type safety.
Let's get going.
Run the codegen to automatically generate types:
_10npm run codegen -w
Next, we'll combine the graphql()
function with the useQuery()
and GraphQL Request hook to fetch the data.
_43import { useMutation, useQuery } from '@apollo/client'_43_43import { graphql } from '../gql/gql'_43_43const GET_TASKS = graphql(`_43 query GetTasks {_43 tasks(order_by: { createdAt: desc }) {_43 id_43 name_43 done_43 }_43 }_43`)_43_43export function Tasks() {_43 const { data, loading, error } = useQuery(GET_TASKS)_43_43 if (loading) {_43 return <div>Loading...</div>_43 }_43_43 if (error) {_43 console.error(error)_43 return <div>Error</div>_43 }_43_43 if (!data) {_43 return <div>No data</div>_43 }_43_43 const { tasks } = data_43_43 return (_43 <div>_43 <h2>Todos</h2>_43 <ul>_43 {tasks.map((task) => (_43 <li key={task.id}>{task.name}</li>_43 ))}_43 </ul>_43 </div>_43 )_43}
That's it. In this scenario, using Nhost, you now have type-safety from Postgres, to GraphQL to TypeScript. From your database to your frontend. Good job!
Now, let's see some benefits of this setup!
If you're trying to access a property on tasks
that does not exist, TypeScript will throw an error. E.g. if you're trying to access task.age
.
_10src/components/Tasks.tsx:160:44 - error TS2339: Property 'age' does not exist on type '{ __typename?: "tasks" | undefined; id: any; name: string; done: boolean; }'._10_10160 <div className={style}>{task.age}</div>_10 ~~~_10_10_10Found 1 error in src/components/Tasks.tsx:160
NOTE: If you're using Vite remember that Vite does not perform type checking. You need to run
tsc -noEmit
to see any TypeScript errors.
If you're using VS Code you also see the TypeScript error while you're coding:
VS Code shows TypeScript errors
TypeScript also knows that task.name
is of type string
, which it has inferred from Postgres ( the task.name
column is of type text
) and GraphQL (task.name
is of type String
). This means you can't use task.name
to perform arithmetic operations.
VS Code shows another TypeScript error.
As you see, with the GraphQL Code Generator (and Postgres, GraphQL, and TypeScript) you're less likely to run into type errors. Overall, your application becomes more robust with fewer bugs.
Let's continue and see how to use GraphQL Code Generator with GraphQL mutations.
Insert Task (Mutation)
Specify the GraphQL mutation and wrap it around the graphql()
function.
Next, specify the mutation in the useMutation
hook. The mutationFn
is the function that will be called when the mutation is triggered. If the mutation is successful, the onSuccess
function will be called where we invalidate the tasks
query to refetch the data.
Now you can use the insertTask
function to trigger the mutation.
_77import { useState } from 'react'_77import { useMutation, useQuery } from '@apollo/client'_77_77import { graphql } from '../gql/gql'_77_77const INSERT_TASK = graphql(`_77 mutation InsertTask($task: tasks_insert_input!) {_77 insertTasks(objects: [$task]) {_77 affected_rows_77 returning {_77 id_77 name_77 }_77 }_77 }_77`)_77_77export function NewTask() {_77 const [name, setName] = useState('')_77_77 const [insertTask, { loading, error }] = useMutation(INSERT_TASK)_77_77 const handleSubmit = async (e: React.FormEvent<HTMLFormElement>) => {_77 e.preventDefault()_77_77 if (!name) {_77 return_77 }_77_77 try {_77 await insertTask({_77 variables: {_77 task: {_77 name,_77 },_77 },_77 refetchQueries: ['GetTasks'],_77 })_77 setName('')_77 alert('Task added!')_77 } catch (error) {_77 return console.error(error)_77 }_77 }_77_77 return (_77 <div>_77 <h2>New Task</h2>_77 <div>_77 <form onSubmit={handleSubmit} className="space-y-4">_77 <div>_77 <label_77 htmlFor="email"_77 className="block text-sm font-medium text-gray-700"_77 >_77 Todo_77 </label>_77 <div className="mt-1">_77 <input_77 type="text"_77 placeholder="Todo"_77 value={name}_77 onChange={(e) => setName(e.target.value)}_77 />_77 </div>_77 </div>_77 {error && <div>Error: {JSON.stringify(error, null, 2)}</div>}_77 <div>_77 <button type="submit" disabled={loading}>_77 Add_77 </button>_77 </div>_77 </form>_77 </div>_77 </div>_77 )_77}
GitHub Repository
The example code for this blog post is on GitHub - GraphQL Code Generator Example with Apollo Client.
Summary
That's it. You've now a basic understanding of how to set up and use GraphQL Code Generator with Apollo Client. This will help you build robust applications with fewer bugs. Good luck!
What's next?
Did you enjoy this blog post?